Implementing a Self-Learning Algorithm for Annealing Prediction
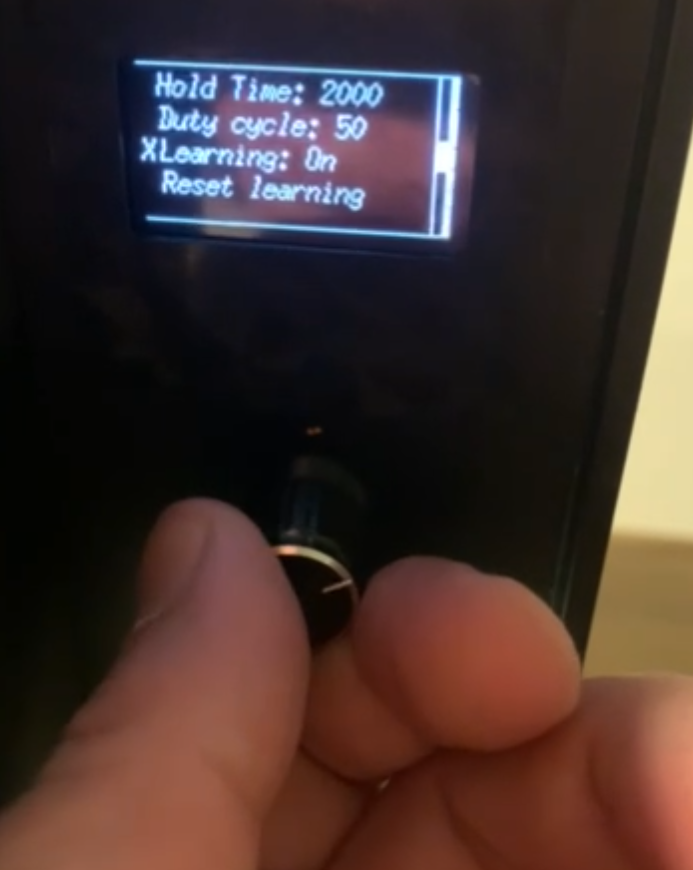
In my previous post on case analysis and prediction of proper annealing, we uncovered the fascinating insight that the current at the desired annealed state follows a linear function of the initial current drawn by the ZVS board at the beginning of an annealing cycle. Now, let’s explore how we can implement a self-learning algorithm in the microcontroller to adapt this function dynamically.
Traditionally, we would derive the function through linear regression on the entire dataset. However, considering the limited storage capacity of microcontrollers, we’ll explore an alternative method: iterative regression analysis.
The predictive function, following the formula y = ax + b, can be modified iteratively using a more efficient data storage approach. Instead of storing individual data points consisting of two current measurements, we only need to store the number of data points (n), mean x (meanGlowCurrentX), mean y (meanGlowCurrentY), variance of x (varXGlowCurrent), and covariance of x and y (covXYGlowCurrent).
Here’s an example of how the resulting function can be updated using an iterative approach:
void updateGlowCurrentFormula() {
nGlowCurrent++;
float dx = baseCurrent - meanGlowCurrentX;
float dy = holdCurrent - meanGlowCurrentY;
varXGlowCurrent += ((float(nGlowCurrent-1)/float(nGlowCurrent))*dx*dx - varXGlowCurrent)/float(nGlowCurrent);
covXYGlowCurrent += (((float(nGlowCurrent-1))/float(nGlowCurrent))*dx*dy - covXYGlowCurrent)/float(nGlowCurrent);
meanGlowCurrentX += dx/float(nGlowCurrent);
meanGlowCurrentY += dy/float(nGlowCurrent);
glowCurrentCoefficient = covXYGlowCurrent/varXGlowCurrent;
glowCurrentConstant = meanGlowCurrentY - glowCurrentCoefficient*meanGlowCurrentX;
}
In this code snippet, the variables nGlowCurrent, baseCurrent, holdCurrent, meanGlowCurrentX, meanGlowCurrentY, varXGlowCurrent, covXYGlowCurrent, glowCurrentCoefficient, and glowCurrentConstant are utilized to update the function iteratively.
By employing this approach, we can dynamically adjust the glowCurrentCoefficient and glowCurrentConstant values every time we provide new current measurements. This allows the microcontroller to continuously learn and adapt its predictive capability based on the evolving dataset.